1. 具体需求
学校院系展示:
编写程序展示一个学校院系结构:要在一个页面中展示出学校的院系组成,一个学校有多个学院,一个学院多有个系,如图。
2. 传统解决方式
1)类图(继承关系)
2)问题分析
- 继承关系,将学院看作是学校的子类,系是学院的子类,这样实际上是站在组织大小来进行分层次的
- 实际上我们的要求是:在一个页面中展示出学校的院系组成,一个学校有多个学院,一个学院有多个系,因此这种方案,不能很好的实现管理的操作,比如对学院、系的增删改查等
- 解决方案:把学校、学院、系都看作是组织结构,他们之间没有继承关系,而是一种树形结构,可以更好的实现管理操作——>组合模式
3. 组合模式解决方式
3.1 基本介绍
1)组合模式(Composite Pattern),又叫部分整体模式,它创建了对象组的树形结构,将对象组合成树状结构以表示“整体-部分”的层次关系。
2)组合模式依据树形结构来组合对象,用来表示部分以及整体层次。
3)这种类型的设计模式属于结构型模式。
4)组合模式使得用户对单个对象和组合对象的访问具有一致性,即:组合能让客户以一致的方式处理个别对象以及组合对象。
3.2 原理及角色
1)类图
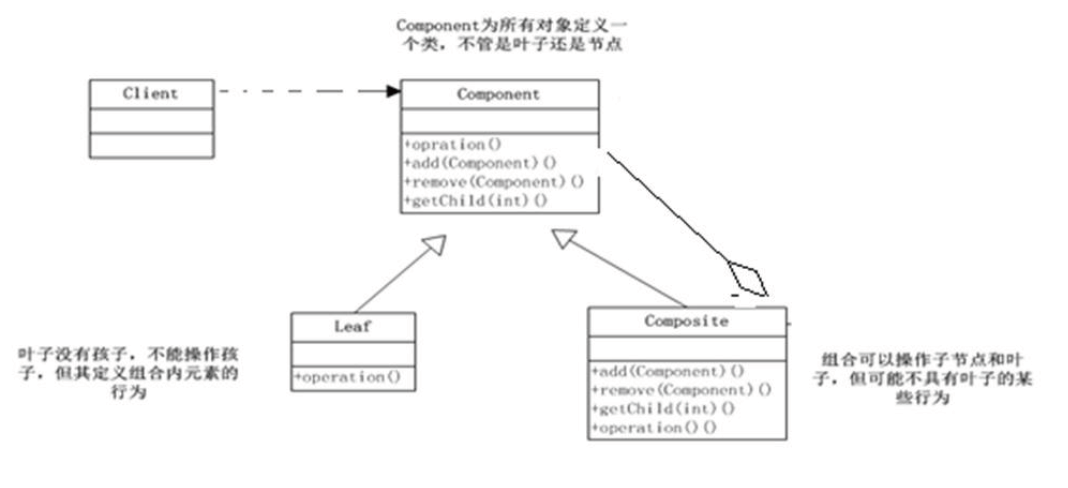
2)角色及职责
- Component:这是组合中对象声明接口,在适当情况下,实现所有类共有的接口默认行为,用于访问和管理Component子部件(子类),Component可以是抽象类或者接口。
- Leaf:在组合中表示叶子节点,叶子节点没有子节点(被管理)
- Composite:非叶子节点,用于存储子部件,在Component接口中实现子部件的相关操作,比如增加(add)、删除……(管理者)
3)组合模式解决的问题
组合模式解决这样的问题,当我们要处理的对象可以生成一颗树形结构,而我们要对树上的节点和叶子节点进行操作时,它能够提供一致的方式,而不用考虑它是节点还是叶子
示意图
3.3 应用实例
用组合模式实现学校院系展示
1)思路分析
2)代码示例
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40//Component
public abstract class OrganizationComponent {
private String name;
private String des;
public OrganizationComponent(String name, String des) {
this.name = name;
this.des = des;
}
protected void add(OrganizationComponent component){
//默认实现
//为什么不写成抽象?因为有一些子类不需要实现所有方法
throw new UnsupportedOperationException();
}
protected void remove(OrganizationComponent component){
//默认实现
//为什么不写成抽象?因为有一些子类不需要实现所有方法
throw new UnsupportedOperationException();
}
protected abstract void print();
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDes() {
return des;
}
public void setDes(String des) {
this.des = des;
}
}
1 | //学校,非叶子节点Composite,可以管理College |
1 | //学院,非叶子节点Composite |
1 | //系,叶子节点,Leaf |
1 | //Test |
4. 组合模式在JDK集合的源码分析
1)Java的集合类-HashMap就使用了组合模式
做了两层Component:Map是一个接口,有put、putAll等方法,而AbstractMap是一个抽象类默认实现Map的方法,
HashMap是非叶子节点Composite,重写了方法
HashMap类中的内部类Node就是叶子节点
2)代码分析+Debug源码
3)类图
5. 组合模式的注意事项和细节
1)简化客户端操作。客户端只需要面对一致的对象而不用考虑整体部分或者节点叶子的问题。 2) 具有较强的扩展性。当我们要更改组合对象时,我们只需要调整内部的层次关系,客户端不用做出任何改动(OCP原则) 3) 方便创建出复杂的层次结构。客户端不用理会组合里面的组成细节,容易添加节点或者叶子从而创建出复杂的树形结构 4) 需要遍历组织机构,或者处理的对象具有树形结构时,非常适合使用组合模式 5) 要求较高的抽象性,如果节点和叶子有很多差异性的话,比如很多方法和属性都不一样,不适合使用组合模式